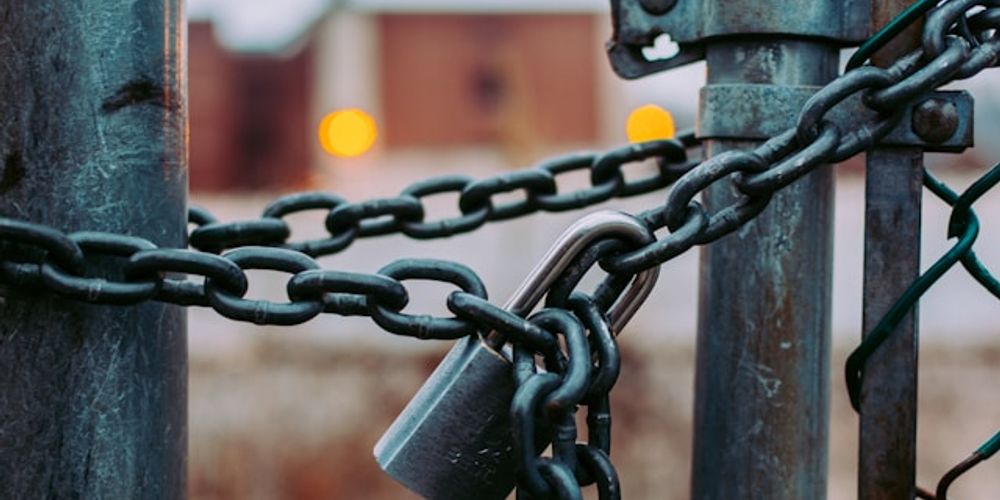
Photo by Jose Fontano
Lock It Down: 3 Keys to Securing Your REST API
REST (Representational State Transfer) APIs have become ubiquitous in modern web and mobile application development, providing a way for different software systems to communicate and share data over the internet. However, the open nature of REST APIs also presents security risks that must be carefully managed.
REST APIs enable applications to access server-side resources using simple HTTP requests. A client sends a request to a server, and the server returns a response, typically in JSON or XML format. But this open access can allow malicious actors to steal, modify, or delete data if proper protections are not in place.
Securing REST APIs involves implementing controls around authentication, authorization, encryption, input validation, rate limiting, monitoring, and more. This article will provide practical guidance and code examples to help API developers and architects build secure interfaces that protect sensitive resources and data.
Topics covered include:
- Authentication: Verifying a user’s identity
- Authorization: Controlling what users can access
- Encryption: Protecting data in transit and at rest
- Input validation: Sanitizing user input
- Rate limiting: Preventing abuse and denial of service
- Logging & monitoring: Tracking activity and detecting attacks
- Security testing: Identifying vulnerabilities
- Documentation & policies: Setting security requirements
By properly implementing these controls, companies can confidently build REST APIs that enable new products and services without compromising security. This article aims to help readers understand REST API risks, make informed decisions, and implement industry standard security measures.
Authentication
Authentication is one of the most critical aspects of API security. It determines how your API verifies and validates the identity of consumers trying to access your data and services. There are several authentication methods commonly used for REST APIs:
-
API Keys - A unique identifier issued to the client to access the API. The client passes the API key as a request parameter or header. API keys are easy to implement but lack advanced security controls.
-
OAuth - An authorization framework that enables delegated access, allowing users to grant a third-party application access to their data on a service provider site without exposing their credentials. It provides selective access and encryption capabilities.
-
JSON Web Tokens (JWT) - A JSON-formatted token that asserts claims about a user identity and permissions. The token is cryptographically signed to prevent tampering. JWT enables single sign-on (SSO) and is stateless once issued.
Some other authentication considerations for REST APIs include:
-
Single Sign-On (SSO) - Allow users to authenticate once with a single ID across multiple applications and services. This improves ease-of-use.
-
Multi-Factor Authentication (MFA) - Require users to provide multiple proofs of identity before granting access, such as a password plus a one-time code sent to their phone. MFA provides an extra layer of security.
-
OAuth 2.0 - Provides authorization flows for web, mobile, and JavaScript apps. It enables SSO and delegation of access without sharing user credentials.
-
OpenID Connect - An identity layer built on OAuth 2.0 that allows clients to verify user identity via authentication providers. Enables SSO across services.
Proper authentication is crucial to ensure only authorized clients can access your API’s resources and prevent exploitation of your services. Evaluate your security requirements to determine the right authentication methods.
Authorization
Authorization refers to rules that determine who is allowed to do what within an API. Proper authorization ensures users can only access resources and perform actions they are permitted to. There are two main authorization strategies for APIs:
Role-Based Access Control (RBAC)
With RBAC, permissions are assigned to roles rather than individual users. For example, you may have a “reader” role that can only retrieve data, an “author” role that can create and edit data, and an “admin” role with full access. When users authenticate, they are assigned a role which dictates their level of access.
Attribute-Based Access Control (ABAC)
ABAC uses attributes about the user, resource, and context to determine access. For example, users may only be allowed to edit resources they created. Or certain resources may only be accessible from approved IP addresses. Policies combine attributes to dynamically make authorization decisions.
Common Authorization Mistakes
-
No authorization - All users have full access. This lacks even basic protection.
-
Not limiting roles - Having roles that are too broad (e.g. “admin”) results in overexposed permissions.
-
Hardcoded authorizations - Business logic should determine access, not hardcoded checks. This lacks flexibility.
-
Assuming authentication equals authorization - Don’t grant access just because a user is logged in.
-
Overly complex rules - While flexibility is good, complex rules can make debugging difficult. Find a balance.
Properly implementing authorization prevents data exposure and ensures users only have the access they require. Role-based and attribute-based access control provide standardized authorization solutions.
Encryption
Encrypting data is crucial for securing REST APIs. There are two main types of encryption to consider:
Encryption of Data in Transit
When data is transmitted between the client and server, it is vulnerable to interception and tampering. REST APIs should use HTTPS with TLS encryption to encrypt all data in transit.
HTTPS uses SSL/TLS protocols to provide a secure connection over the internet. The data is encrypted before sending and decrypted after receiving. This protects the confidentiality and integrity of the data while it travels across the network.
Using HTTPS ensures that eavesdroppers cannot read or modify the data during transmission. It also verifies the identity of the API server through certificates to prevent man-in-the-middle attacks.
Encryption of Data at Rest
In addition to encrypting data in transit, sensitive data should also be encrypted when stored at rest on servers. This protects the data if the servers are compromised.
Common strategies include encrypting databases, digitally encrypting sensitive fields or columns before storage, and using encrypted filesystems. The encryption keys should be securely managed and backed up.
API Request/Response Encryption
For additional security, consider encrypting the entire request and response body of the API, not just the transit. This fully protects the data from unauthorized access.
Encryption at the message level rather than only the transport level provides an extra layer of security. It ensures the data remains encrypted even when briefly buffered or stored inside the API service infrastructure.
The API should allow clients to use public-key cryptography to encrypt requests end-to-end. The server can then decrypt requests using its private key before processing. Responses can be encrypted back to the client in a similar manner.
Input Validation
Protecting against malicious user input is crucial for securing your API. All user-supplied data should be validated and sanitized before processing. Some key input validation strategies include:
-
Sanitizing and validating user input - Scrub all input for invalid characters and truncate/reject overly long input. Whitelist acceptable characters instead of blacklisting prohibited ones. Normalize and encode data into a consistent internal format.
-
Preventing common attacks like SQL injection - Use parameterized queries or an ORM to separate query logic from user-supplied values. This prevents the API from interpreting input as code. Input should be validated against an allowlist of accepted values.
-
Schema validation for requests - Leverage validation schemas like JSON Schema to define structure and constraints for API payloads. Requests should match the schema format and constraints. Custom validation code can supplement schema checks.
Rigorous input validation hardens your API against threats like code injection, protocol manipulation, and unintended data leakage. Defining strict allowlists of acceptable values for each parameter is preferred over trying to blacklist prohibited inputs.
Rate Limiting
Rate limiting is an important technique for preventing denial-of-service (DoS) attacks against your API. By setting appropriate limits on the number of requests clients can make, you can prevent malicious actors from overwhelming your API with traffic and make it unavailable to legitimate users.
There are a few common approaches to implementing rate limiting:
-
IP-based rate limiting - Restrict the number of requests allowed from a specific IP address over a period of time. This prevents a single client from making too many requests. You may also maintain an IP blacklist to block known abusive IPs.
-
User-based rate limiting - If your API uses authentication, you can restrict the rate limits based on user accounts. This prevents any single account from overusing the API.
-
Request limiting - Apply limits to specific endpoints. For example, you may allow 60 requests per minute to the
/search
endpoint but only 15 requests per minute to/purchase
. Restrict APIs that involve more resource intensive operations. -
Global rate limits - Enforce an overarching rate limit on all API requests from an IP or user. This acts as a final layer of protection if other limits are exceeded.
When setting rate limits, balance security and performance. Limits that are too strict may hamper legitimate use cases. Monitor API usage patterns and adjust as needed. Include rate limiting details in your API documentation.
Rate limiting helps prevent API resources from being overused for malicious purposes. With thoughtful implementation, it can enhance API security without severely impacting developers building applications against the API.
Logging & Monitoring
Robust logging and monitoring are key to securing any API. At minimum, you should be tracking all API requests and responses to have visibility into usage patterns. This allows you to detect anomalous activity that could indicate an attack.
Specifically, you should log:
- All API requests and responses, including IP address, user agent, request parameters, response codes, and latency
- User authentication events like logins and failed logins
- Key events like account registrations, password resets, data modifications
Analyze these logs to build a baseline of normal behavior. Anything that deviates, such as a spike in traffic, increase in errors, or activity from a suspicious IP range, should trigger alerts.
Set up monitors and alerts around key API security events like:
- Rate limiting and throttling
- Invalid or expired tokens
- Unrecognized client applications
- Repeated failed login attempts
Log analysis tools like the ELK stack can help visualize patterns and uncover security incidents. Further integrate monitoring with notification services to alert relevant personnel about policy violations or abuse in real time.
Active monitoring allows rapid response to contain an attack before it compromises large amounts of data. Auditing logs regularly also helps identify weaknesses and enhance the overall security posture of the API. Maintaining comprehensive activity records is crucial for forensics if a data breach does occur.
Security Testing
Robust security testing is crucial for identifying vulnerabilities in your API before they can be exploited. There are several recommended methods:
Unit Testing
Unit tests validate that individual components of your API work as intended. They help catch issues early in the development process.
Integration Testing
Integration tests verify that different modules or services work together correctly. They ensure the entire API functions properly.
Penetration Testing
Penetration testing involves simulating attacks to probe for vulnerabilities. Ethical hackers use tools and techniques that real attackers would use.
Automated Scanners
Static and dynamic analysis tools can automatically scan your API codebase to uncover security flaws. They complement manual testing.
Bug Bounty Programs
Bug bounties incentivize security researchers to find and report vulnerabilities. They help discover issues that may have been missed.
Overall, utilizing multiple testing methods provides defense in depth. Prioritize testing critical authentication, authorization, and data handling functions. Perform testing regularly, especially after making code changes. Find and remediate vulnerabilities before releasing your API.
Documentation & Policies
Clear and comprehensive API documentation is crucial for proper API security. The documentation should describe all endpoints, request and response formats, authentication methods, rate limits, and any other usage constraints.
Developers should not have to guess how to properly use the API. The documentation should provide examples of requests and responses to demonstrate proper usage.
Well-defined usage terms and conditions should be made available to developers. This establishes what is and is not permitted when using the API. For example, are there restrictions on number of requests, data usage caps, etc? This avoids misunderstandings down the road.
A security vulnerability disclosure policy outlines the proper process for security researchers and users to report any vulnerabilities they discover. It should provide clear instructions for making confidential disclosure to your security team and establish your commitment to investigating and addressing reported issues appropriately rather than threatening legal action. Such policies help facilitate crowdsourced auditing of your API security.
With clear documentation, defined usage terms, and a responsible vulnerability disclosure policy, developers will understand how to properly use your API and help you identify any potential weaknesses. This promotes correct API usage and better security for your data.
Conclusion
When developing and hosting REST APIs, security should be a top priority. The data transmitted through APIs often contains sensitive information that could enable fraud, identity theft, and data breaches if accessed by attackers.
The strategies covered in this article, when used together, create a layered security approach that makes it extremely difficult for unauthorized parties to compromise your APIs. Authentication verifies user identities, authorization controls access, and encryption protects data while in transit and at rest. Input validation, rate limiting, robust logging and monitoring, and comprehensive security testing also help lock down APIs.
Adopting these security best practices enables companies to protect customer data, safeguard intellectual property, and maintain compliance with regulations like GDPR and PCI DSS. The effort required to properly secure APIs pays dividends by preventing devastating cyber attacks, data leaks, and privacy violations.
The security of APIs directly impacts user trust and the reputation of API providers. Investing resources into API security demonstrates respect for customers and a commitment to protecting their information. With cyber threats growing in scale and sophistication, proper API security has never been more crucial.
Stay tuned with APIRobots for more insights and updates on this exciting field. Don’t miss out on the opportunities that APIs can bring to your business. Contact us today at API Robots an APIs Development Agency and let’s unlock the full potential of APIs together.