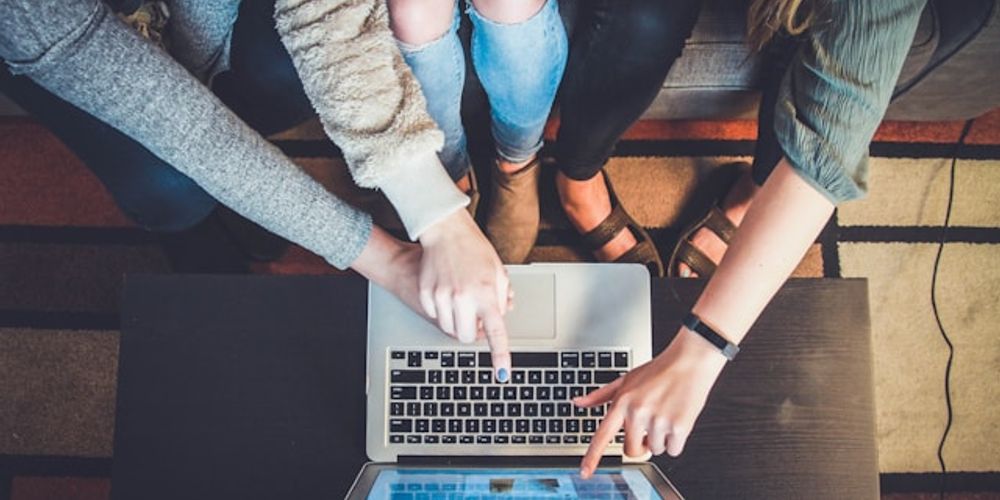
Photo by John Schnobrich
The Ultimate Guide to Automated Testing for REST APIs
REST (Representational State Transfer) APIs have become the de facto standard for building APIs and web services. REST APIs provide a simple, lightweight and scalable architecture for accessing resources over HTTP.
With REST APIs, each resource has a unique URL that represents a specific request to get or modify data. REST uses standard HTTP methods like GET, POST, PUT, PATCH, and DELETE to perform operations on these resources. JSON is commonly used as the data exchange format to provide a human-readable structure.
REST APIs have gained immense popularity in recent years due to the following key advantages:
-
Flexibility - REST APIs can be accessed by any device or client that can make HTTP requests. This makes them platform and language agnostic.
-
Performance - REST APIs provide fast performance and scalability as there is no strict processing pipeline. Less bandwidth is used compared to heavy protocols like SOAP.
-
Caching - HTTP caching features can be utilized to improve performance. Responses can be cached to avoid duplicate requests.
-
Loose coupling - The client and server are loosely coupled, allowing more independent evolution. Changes to the API or implementation only affect the interface.
-
Scalability - REST APIs scale well horizontally as REST calls can be load balanced across multiple servers. Less network traffic and straight-forward implementation enable scalability.
As REST APIs become central to many data-driven applications today, comprehensive testing and validation are crucial to ensure correctness and reliability. Proper automated testing provides confidence that the API meets requirements specifications and performs as intended under various conditions. This guide covers best practices and techniques for effective automated testing of REST APIs.
Benefits of Testing REST APIs
Testing REST APIs provides significant benefits that make the effort worthwhile. Thorough testing of REST APIs can help:
-
Find bugs early in the development process. Testing REST API endpoints as they are built enables developers to catch issues while they are still small and easy to fix. This prevents bugs from persisting and multiplying throughout the code.
-
Reduce defects in production. Comprehensive testing before deployment helps minimize the defects that make it to production systems. This improves the end user experience and saves time fixing issues after the fact.
-
Ensure reliability and stability. Testing confirms the REST API works as expected, even with different parameters and payloads. Rigorous testing ensures the API produces the right outputs and error handling for a wide array of requests.
-
Give confidence to make changes. Refactoring and modifying the API is done with less concern when thorough tests are in place. Automated tests can be run to verify changes did not break existing functionality.
-
Meet specifications and requirements. Tests derived from specifications give assurance that the implementation meets acceptance criteria. Issues are found quickly if the REST API does not satisfy functional requirements.
In summary, taking the effort to adequately test REST APIs provides confidence in the code, reduces future maintenance costs, and leads to more robust and high quality APIs. The benefits of preventing bugs from ever reaching production systems makes the effort of comprehensive API testing worthwhile.
Types of Testing for REST APIs
When creating a robust testing strategy for REST APIs, there are several types of testing that should be included:
Unit Testing
Unit testing focuses on testing individual units or components of the API codebase, such as functions or classes. The goal is to validate that each unit operates as intended in isolation from the rest of the system. Unit testing helps catch bugs early in the development cycle.
Integration Testing
Integration testing verifies that different modules or services of an API work together as expected. Testing end-to-end API workflows rather than individual units is the focus. Common integration testing approaches include testing routes and endpoints, making actual API calls, testing authentication, and confirming integration with databases and other external systems.
System Testing
System testing looks at the API as a whole from an end-user perspective. The entire integrated system is tested to validate full system functionality and conformance to requirements. Black box testing methods that examine inputs and outputs without looking at internal code are often used.
Regression Testing
Existing and new tests are run every time the API is modified to catch any regressions where something previously functioning correctly starts failing. Running automated regression test suites helps maintain software quality over time.
Load Testing
Load tests place demand on the API to validate performance levels under different user loads. Testing at scale helps identify bottlenecks, slow endpoints, and resource issues before launch.
Security Testing
Various techniques are used to validate the API’s ability to withstand security threats like cross-site scripting, SQL injection, spoofing, brute force attacks, etc. Security testing identifies vulnerabilities a malicious actor could potentially exploit.
Compatibility Testing
Compatibility testing verifies consistent functionality across different operating systems, browsers, and devices. REST APIs should perform reliably regardless of client platforms. Automated testing across multiple environments is recommended.
Thoroughly testing REST APIs reduces bugs and errors, improves reliability and performance, and ensures a smooth user experience. The above testing types each play an important role in the overall testing strategy.
Unit Testing REST APIs
Unit testing validates individual parts of the system, such as API endpoints, to verify they function as expected. With REST APIs, unit tests should focus on testing:
-
Individual endpoints and operations - Each endpoint should be tested independently to ensure it handles expected requests properly. Test common operations like GET, POST, PUT, DELETE.
-
Status codes - Validate that the API returns the appropriate HTTP status codes for different request scenarios. For example, a 200 OK for a successful GET request, 404 Not Found for a non-existent resource, etc.
-
Request/response schemas - The request and response payloads should conform to the defined schema for that endpoint. Unit tests can validate the payload structure, required fields, data types, etc.
-
Edge cases - Test edge cases that could break the endpoint, such as missing required parameters, invalid data formats, special characters, rate limits exceeded, etc.
Unit testing REST APIs gives confidence that each endpoint works as intended before integrating them together. It helps catch bugs early and makes it easier to pinpoint issues. Frameworks like JUnit and Mocha provide a simple way to write and manage API unit tests using assertions.
Integration Testing REST APIs
Integration testing focuses on verifying how different parts of an API work together as a whole. The key aspects of integration testing REST APIs include:
-
Testing interactions between multiple endpoints - While unit tests focus on individual endpoints, integration tests validate how endpoints work together in a sequence. This involves testing workflows and user journeys that span multiple endpoints.
-
Testing dependencies between endpoints - APIs often have dependencies where one endpoint relies on data from another endpoint. Integration tests validate these dependencies by seeding test data and asserting outcomes across endpoints.
-
Testing data flow across endpoints - Data created via one endpoint often needs to be consumed by other endpoints. Integration tests verify the consistency and accuracy of data as it flows through a series of API calls.
-
Testing side effects of endpoint interactions - In addition to the direct outcomes, integration tests also check for side effects resulting from sequences of API calls like changes to databases, external systems, etc.
-
Testing error handling across endpoints - Integration tests validate how errors bubble up through chains of endpoint requests and verify if error handling is consistent.
-
End-to-end testing of the entire API - Larger integration tests act as end-to-end tests by exercising the full API functionality from start to finish.
Effective integration testing requires simulating real-world API usage by calling multiple endpoints in logical workflows. Testing only individual endpoints in isolation is not enough to catch integration issues. Automated testing tools and mocking help create and run these complex integration test scenarios.
System Testing REST APIs
System testing validates the complete API system from end to end. It focuses on verifying that the integrated API meets all requirements and specified behaviors.
System testing exercises the full API implementation, including the backend services and databases it connects to. It tests APIs “black box” based solely on their specifications.
Some key aspects of system testing REST APIs include:
-
Test API functionality with different combinations of valid and invalid inputs. Verify the API produces the expected errors and appropriate status codes.
-
Ensure the API performs correctly under different conditions such as various user roles and permissions. Test with proper auth tokens and without.
-
Validate that the API works as expected when connecting to integrated systems. Test calls when backend services are online or offline.
-
Load test the API with varying user loads. Check for performance, response times, and scalability issues.
-
Test all mainline and alternate flows through the API. Execute positive, negative, exception, and edge case tests.
-
Validate that the API handles large payloads and different content types like XML and JSON properly.
-
Monitor API logs during testing. Verify that errors and requests are being logged correctly.
-
Run tests across different environments like dev, QA, and staging. Check for configuration issues.
-
Automate system tests so they can be rerun quickly against new releases and as part of regression testing.
Performing comprehensive system testing against REST APIs helps ensure they are robust and production-ready before release. Testers validate end-to-end functionality and integration points under real-world conditions.
Automated API Testing Tools
There are many useful tools available for automated testing of REST APIs. Here are some of the most popular options:
Postman
Postman is one of the most widely used API testing tools. It provides an intuitive GUI for constructing requests and reading responses. Some key features include:
- Building and saving requests for later use
- Automatic code snippet generation for languages like JavaScript and Python
- Customizable test scripts to validate responses
- Collection runner to perform regression testing
- Built-in documentation for APIs
Overall, Postman makes it very easy to get started with API testing without needing to write code.
Karate
Karate is an open-source API test automation framework that uses Cucumber-style Gherkin syntax. It allows writing test cases in a readable domain-specific language. Key features of Karate include:
- Ability to script API tests and leave no step untested
- Re-usability of test scripts across protocols like HTTP, SOAP, Kafka etc.
- Dynamic Scenario Outline syntax for data-driven testing
- Support for performance testing with Karate Gatling
- Seamless integration with CI/CD workflows
Karate is designed for testing professionals and developers seeking more flexibility.
REST Assured
REST Assured is a Java library that simplifies testing and validating REST services. Its key strengths are:
- Natural language for assertions
- Extensive support for request and response specifications
- Seamless integration with testing frameworks like JUnit and TestNG
- Flexible JSON and XML response parsing
- Support for different authentication mechanisms
- Ability to validate status codes, headers, cookies etc.
For Java developers building REST APIs, REST Assured is a great choice with rich features.
JMeter
JMeter is an open source, Java-based load and performance testing tool. For REST APIs, its capabilities include:
- Recording HTTP requests and building test plans
- Load testing to simulate multiple concurrent users
- Graphical analysis and custom reporting of results
- Creating scripts with JSR223 elements and Groovy code
- Distributed testing by coordinating multiple JMeter instances
- Plug-in support to enhance core features
JMeter allows thoroughly testing the reliability and scalability of REST APIs.
SoapUI
SoapUI is a functional testing tool used mainly for SOAP web services. However, it also has extensive support for REST APIs:
- Configuring REST requests quickly via its GUI
- Asserting the contents of responses
- Scripting with Groovy for greater control
- Simulating message exchanges between services
- Generating automated test cases and mock services
- Integrations with CI tools like Jenkins
SoapUI provides a feature-rich option for testing REST and SOAP-based web services.
There are ample tools available for automating various aspects of REST API testing. The needs of the API and the team will determine which option is best.
API Mocking
Mocking is an important technique for testing REST APIs. It involves simulating API endpoints to mimic the behavior of a real API. Using mocks allows you to test your API client code without having to rely on the actual backend implementation.
There are several benefits to mocking APIs during testing:
-
Avoid dependencies on external systems. Mocks allow you to test in isolation without needing a real API backend. This speeds up testing and avoids flakiness when external dependencies change.
-
Test edge cases. Using mocks makes it easy to simulate responses for unusual scenarios like error states. It’s hard to force a real API to return errors on demand.
-
Isolate failures. If a test fails you can be sure the problem is in your client code and not the backend API. Mocks remove the API as a scapegoat.
-
Work offline. Mocks allow testing without an internet connection to the real API servers.
There are two main approaches to mocking APIs:
-
Mock web server - This uses a local web server to respond to API requests during testing. Requests are intercepted and stubs are used to simulate responses. Popular tools include WireMock, MockServer and Mountebank.
-
Mock client - Libraries like Mockito can be used to mock the HTTP client directly rather than mocking the server. The client can be configured to return stubbed responses.
Overall mocking is an essential technique for robust automated testing of REST APIs. It removes external dependencies and allows you to thoroughly test edge cases. Using mocks provides fast, reliable tests that give you confidence in your API client code.
Best Practices for Automated API Testing
To ensure your automated API tests are effective and maintainable, follow these best practices:
Maintainable and Reusable Tests
-
Modularize tests into smaller functions that can be combined into test suites. This makes tests more maintainable and reusable across projects.
-
Avoid hardcoding values like URLs, credentials, or test data. Instead, store these in configuration files that can be imported.
-
Name tests clearly and consistently to make them easy to understand. Group related tests into test suites.
-
Refactor tests when endpoints change rather than rewriting from scratch. Extract common logic into utility functions.
Proper Setup and Teardown
-
Establish test environments, datasets, and API state before running tests. Reset these after tests complete.
-
Use test hooks or utilities to handle repeated setup/teardown tasks like generating test data or clearing databases.
-
Ensure proper authentication is handled in setup rather than in each test.
Monitoring and Logging
-
Log key info like API requests/responses, failures, and pass/fail status to simplify debugging.
-
Use a test runner that shows test progress, summaries, and historical testing trends.
-
Monitor tests in CI/CD pipelines to catch failures immediately. Rerun tests periodically.
-
Integrate tests with monitoring tools to track test coverage over time.
Following these best practices will optimize your automated API tests for reliability, reusability, and maintainability.
Conclusion
Automated testing is critical for developing and maintaining reliable REST APIs. As discussed throughout this guide, thorough testing at the unit, integration, and system levels helps catch bugs and issues early before they impact end users. It also ensures the API endpoints consistently meet requirements and specifications.
By leveraging test automation tools and frameworks like Postman, Rest Assured, and Mockito, teams can significantly accelerate testing cycles. This enables faster delivery of high-quality APIs. With the right testing strategy and processes in place, developers can ship new API features and enhancements frequently and confidently.
To summarize, investing in comprehensive API testing automation provides manifold benefits:
- Reduces bugs in production that cause application failures
- Catches edge cases and errors that may not be found through manual testing alone
- Validates that integrations with frontends/clients work as expected
- Gives developers confidence to release frequently
- Facilitates test driven development of new features
- Prevents regressions when the API is modified
- Improves reliability and stability of the API
As APIs continue to proliferate and serve as the integration backbone for microservices and distributed systems, having robust automation for API testing is no longer optional. It is a prerequisite for developing world-class APIs that exceed customer expectations. This guide has provided a blueprint for getting started with and implementing API testing practices that will result in higher quality and more resilient REST APIs.
Stay tuned with APIRobots for more insights and updates on this exciting field. Don’t miss out on the opportunities that APIs can bring to your business. Contact us today at API Robots an API First Development Agency and let’s unlock the full potential of APIs together.